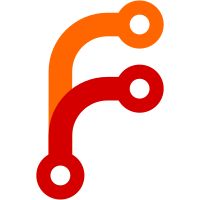
Up until now the julian package relied on the time.Time type to represent Gregorian dates, and julian.Date to represent Julian dates, however this both looks inconsistent, and is more opaque and complicated because time.Time carries with it a lot of information we don't care about. Therefore this has been modified, now the conversion package has two types julian.JDate and julian.GDate for their respective calendars.
221 lines
9.3 KiB
Go
221 lines
9.3 KiB
Go
package julian
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func TestMonthLength(t *testing.T) {
|
|
var tests = []struct {
|
|
y int
|
|
m time.Month
|
|
want int
|
|
}{
|
|
{2001, time.January, 31},
|
|
{2001, time.February, 28},
|
|
{2000, time.February, 29},
|
|
{2001, time.March, 31},
|
|
{2001, time.April, 30},
|
|
{2001, time.May, 31},
|
|
{2001, time.June, 30},
|
|
{2001, time.July, 31},
|
|
{2001, time.August, 31},
|
|
{2001, time.September, 30},
|
|
{2001, time.October, 31},
|
|
{2001, time.November, 30},
|
|
{2001, time.December, 31},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
testname := fmt.Sprintf("%v, %d", tt.m, tt.y)
|
|
t.Run(testname, func(t *testing.T) {
|
|
ans := monthLength(tt.y, tt.m)
|
|
if ans != tt.want {
|
|
t.Errorf("got %d, want %d", ans, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestJDateDiff(t *testing.T) {
|
|
var tests = []struct {
|
|
d JDate
|
|
days int
|
|
want JDate
|
|
}{
|
|
{JDate{2001, time.January, 20}, 5, JDate{2001, time.January, 25}},
|
|
{JDate{2001, time.February, 28}, 5, JDate{2001, time.March, 5}},
|
|
{JDate{2004, time.February, 28}, 5, JDate{2004, time.March, 4}},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
testname := fmt.Sprintf("%v, %d", tt.d, tt.days)
|
|
t.Run(testname, func(t *testing.T) {
|
|
ans := jDateDiff(tt.d, tt.days)
|
|
if ans != tt.want {
|
|
t.Errorf("got %d, want %d", ans, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestNextMonth(t *testing.T) {
|
|
ans1, ans2 := nextMonth(2000, time.December)
|
|
if ans1 != 2001 || ans2 != time.January {
|
|
t.Errorf("nextMonth(2000, time.December) = %d, %v; want 2001, time.January", ans1, ans2)
|
|
}
|
|
}
|
|
|
|
func TestJDateToGDate(t *testing.T) {
|
|
var tests = []struct {
|
|
d JDate
|
|
want GDate
|
|
}{
|
|
{JDate{100, time.February, 29}, GDate{100, time.February, 27}},
|
|
{JDate{100, time.March, 1}, GDate{100, time.February, 28}},
|
|
{JDate{100, time.March, 2}, GDate{100, time.March, 1}},
|
|
{JDate{200, time.February, 28}, GDate{200, time.February, 27}},
|
|
{JDate{200, time.February, 29}, GDate{200, time.February, 28}},
|
|
{JDate{200, time.March, 1}, GDate{200, time.March, 1}},
|
|
{JDate{300, time.February, 28}, GDate{300, time.February, 28}},
|
|
{JDate{300, time.February, 29}, GDate{300, time.March, 1}},
|
|
{JDate{300, time.March, 1}, GDate{300, time.March, 2}},
|
|
{JDate{500, time.February, 28}, GDate{500, time.March, 1}},
|
|
{JDate{500, time.February, 29}, GDate{500, time.March, 2}},
|
|
{JDate{500, time.March, 1}, GDate{500, time.March, 3}},
|
|
{JDate{600, time.February, 28}, GDate{600, time.March, 2}},
|
|
{JDate{600, time.February, 29}, GDate{600, time.March, 3}},
|
|
{JDate{600, time.March, 1}, GDate{600, time.March, 4}},
|
|
{JDate{700, time.February, 28}, GDate{700, time.March, 3}},
|
|
{JDate{700, time.February, 29}, GDate{700, time.March, 4}},
|
|
{JDate{700, time.March, 1}, GDate{700, time.March, 5}},
|
|
{JDate{900, time.February, 28}, GDate{900, time.March, 4}},
|
|
{JDate{900, time.February, 29}, GDate{900, time.March, 5}},
|
|
{JDate{900, time.March, 1}, GDate{900, time.March, 6}},
|
|
{JDate{1000, time.February, 28}, GDate{1000, time.March, 5}},
|
|
{JDate{1000, time.February, 29}, GDate{1000, time.March, 6}},
|
|
{JDate{1000, time.March, 1}, GDate{1000, time.March, 7}},
|
|
{JDate{1100, time.February, 28}, GDate{1100, time.March, 6}},
|
|
{JDate{1100, time.February, 29}, GDate{1100, time.March, 7}},
|
|
{JDate{1100, time.March, 1}, GDate{1100, time.March, 8}},
|
|
{JDate{1300, time.February, 28}, GDate{1300, time.March, 7}},
|
|
{JDate{1300, time.February, 29}, GDate{1300, time.March, 8}},
|
|
{JDate{1300, time.March, 1}, GDate{1300, time.March, 9}},
|
|
{JDate{1400, time.February, 28}, GDate{1400, time.March, 8}},
|
|
{JDate{1400, time.February, 29}, GDate{1400, time.March, 9}},
|
|
{JDate{1400, time.March, 1}, GDate{1400, time.March, 10}},
|
|
{JDate{1500, time.February, 28}, GDate{1500, time.March, 9}},
|
|
{JDate{1500, time.February, 29}, GDate{1500, time.March, 10}},
|
|
{JDate{1500, time.March, 1}, GDate{1500, time.March, 11}},
|
|
{JDate{1582, time.October, 4}, GDate{1582, time.October, 14}},
|
|
{JDate{1582, time.October, 5}, GDate{1582, time.October, 15}},
|
|
{JDate{1582, time.October, 6}, GDate{1582, time.October, 16}},
|
|
{JDate{1700, time.February, 18}, GDate{1700, time.February, 28}},
|
|
{JDate{1700, time.February, 19}, GDate{1700, time.March, 1}},
|
|
{JDate{1700, time.February, 28}, GDate{1700, time.March, 10}},
|
|
{JDate{1700, time.February, 29}, GDate{1700, time.March, 11}},
|
|
{JDate{1700, time.March, 1}, GDate{1700, time.March, 12}},
|
|
{JDate{1800, time.February, 17}, GDate{1800, time.February, 28}},
|
|
{JDate{1800, time.February, 18}, GDate{1800, time.March, 1}},
|
|
{JDate{1800, time.February, 28}, GDate{1800, time.March, 11}},
|
|
{JDate{1800, time.February, 29}, GDate{1800, time.March, 12}},
|
|
{JDate{1800, time.March, 1}, GDate{1800, time.March, 13}},
|
|
{JDate{1900, time.February, 16}, GDate{1900, time.February, 28}},
|
|
{JDate{1900, time.February, 17}, GDate{1900, time.March, 1}},
|
|
{JDate{1900, time.February, 28}, GDate{1900, time.March, 12}},
|
|
{JDate{1900, time.February, 29}, GDate{1900, time.March, 13}},
|
|
{JDate{1900, time.March, 1}, GDate{1900, time.March, 14}},
|
|
{JDate{2100, time.February, 15}, GDate{2100, time.February, 28}},
|
|
{JDate{2100, time.February, 16}, GDate{2100, time.March, 1}},
|
|
{JDate{2100, time.February, 28}, GDate{2100, time.March, 13}},
|
|
{JDate{2100, time.February, 29}, GDate{2100, time.March, 14}},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
testname := fmt.Sprintf("%v, %v", tt.d, tt.want)
|
|
t.Run(testname, func(t *testing.T) {
|
|
ans := JDateToGDate(tt.d)
|
|
if ans != tt.want {
|
|
t.Errorf("got %v, want %v", ans, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestGDateToJDate(t *testing.T) {
|
|
var tests = []struct {
|
|
gd GDate
|
|
want JDate
|
|
}{
|
|
{GDate{100, time.February, 27}, JDate{100, time.February, 29}},
|
|
{GDate{100, time.February, 28}, JDate{100, time.March, 1}},
|
|
{GDate{100, time.March, 1}, JDate{100, time.March, 2}},
|
|
{GDate{200, time.February, 27}, JDate{200, time.February, 28}},
|
|
{GDate{200, time.February, 28}, JDate{200, time.February, 29}},
|
|
{GDate{200, time.March, 1}, JDate{200, time.March, 1}},
|
|
{GDate{300, time.February, 28}, JDate{300, time.February, 28}},
|
|
{GDate{300, time.March, 1}, JDate{300, time.February, 29}},
|
|
{GDate{300, time.March, 2}, JDate{300, time.March, 1}},
|
|
{GDate{500, time.March, 1}, JDate{500, time.February, 28}},
|
|
{GDate{500, time.March, 2}, JDate{500, time.February, 29}},
|
|
{GDate{500, time.March, 3}, JDate{500, time.March, 1}},
|
|
{GDate{600, time.March, 2}, JDate{600, time.February, 28}},
|
|
{GDate{600, time.March, 3}, JDate{600, time.February, 29}},
|
|
{GDate{600, time.March, 4}, JDate{600, time.March, 1}},
|
|
{GDate{700, time.March, 3}, JDate{700, time.February, 28}},
|
|
{GDate{700, time.March, 4}, JDate{700, time.February, 29}},
|
|
{GDate{700, time.March, 5}, JDate{700, time.March, 1}},
|
|
{GDate{900, time.March, 4}, JDate{900, time.February, 28}},
|
|
{GDate{900, time.March, 5}, JDate{900, time.February, 29}},
|
|
{GDate{900, time.March, 6}, JDate{900, time.March, 1}},
|
|
{GDate{1000, time.March, 5}, JDate{1000, time.February, 28}},
|
|
{GDate{1000, time.March, 6}, JDate{1000, time.February, 29}},
|
|
{GDate{1000, time.March, 7}, JDate{1000, time.March, 1}},
|
|
{GDate{1100, time.March, 6}, JDate{1100, time.February, 28}},
|
|
{GDate{1100, time.March, 7}, JDate{1100, time.February, 29}},
|
|
{GDate{1100, time.March, 8}, JDate{1100, time.March, 1}},
|
|
{GDate{1300, time.March, 7}, JDate{1300, time.February, 28}},
|
|
{GDate{1300, time.March, 8}, JDate{1300, time.February, 29}},
|
|
{GDate{1300, time.March, 9}, JDate{1300, time.March, 1}},
|
|
{GDate{1400, time.March, 8}, JDate{1400, time.February, 28}},
|
|
{GDate{1400, time.March, 9}, JDate{1400, time.February, 29}},
|
|
{GDate{1400, time.March, 10}, JDate{1400, time.March, 1}},
|
|
{GDate{1500, time.March, 9}, JDate{1500, time.February, 28}},
|
|
{GDate{1500, time.March, 10}, JDate{1500, time.February, 29}},
|
|
{GDate{1500, time.March, 11}, JDate{1500, time.March, 1}},
|
|
{GDate{1582, time.October, 14}, JDate{1582, time.October, 4}},
|
|
{GDate{1582, time.October, 15}, JDate{1582, time.October, 5}},
|
|
{GDate{1582, time.October, 16}, JDate{1582, time.October, 6}},
|
|
{GDate{1700, time.February, 28}, JDate{1700, time.February, 18}},
|
|
{GDate{1700, time.March, 1}, JDate{1700, time.February, 19}},
|
|
{GDate{1700, time.March, 10}, JDate{1700, time.February, 28}},
|
|
{GDate{1700, time.March, 11}, JDate{1700, time.February, 29}},
|
|
{GDate{1700, time.March, 12}, JDate{1700, time.March, 1}},
|
|
{GDate{1800, time.February, 28}, JDate{1800, time.February, 17}},
|
|
{GDate{1800, time.March, 1}, JDate{1800, time.February, 18}},
|
|
{GDate{1800, time.March, 11}, JDate{1800, time.February, 28}},
|
|
{GDate{1800, time.March, 12}, JDate{1800, time.February, 29}},
|
|
{GDate{1800, time.March, 13}, JDate{1800, time.March, 1}},
|
|
{GDate{1900, time.February, 28}, JDate{1900, time.February, 16}},
|
|
{GDate{1900, time.March, 1}, JDate{1900, time.February, 17}},
|
|
{GDate{1900, time.March, 12}, JDate{1900, time.February, 28}},
|
|
{GDate{1900, time.March, 13}, JDate{1900, time.February, 29}},
|
|
{GDate{1900, time.March, 14}, JDate{1900, time.March, 1}},
|
|
{GDate{2100, time.February, 28}, JDate{2100, time.February, 15}},
|
|
{GDate{2100, time.March, 1}, JDate{2100, time.February, 16}},
|
|
{GDate{2100, time.March, 13}, JDate{2100, time.February, 28}},
|
|
{GDate{2100, time.March, 14}, JDate{2100, time.February, 29}},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
testname := fmt.Sprintf("%v, %v", tt.gd, tt.want)
|
|
t.Run(testname, func(t *testing.T) {
|
|
ans := GDateToJDate(tt.gd)
|
|
if ans != tt.want {
|
|
t.Errorf("got %v, want %v", ans, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|