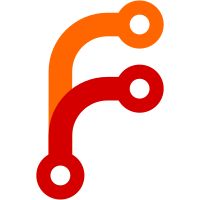
To be noted: the drawing in exercise 1.14 is unfinished. I did it in a notebook, but haven't yet had the time to put it in a txt file.
24 lines
No EOL
513 B
Scheme
24 lines
No EOL
513 B
Scheme
#lang sicp
|
|
|
|
; recursive process
|
|
(define (f1 n)
|
|
(if (< n 3)
|
|
n
|
|
(+ (f1 (- n 1))
|
|
(* 2 (f1 (- n 2)))
|
|
(* 3 (f1 (- n 3))))))
|
|
|
|
; iterative process
|
|
|
|
; loop for n >= 3
|
|
(define (f-iter iter-count acc1 acc2 acc3)
|
|
(let ((new-val (+ acc1 (* 2 acc2) (* 3 acc3))))
|
|
(if (= iter-count 0)
|
|
new-val
|
|
(f-iter (- iter-count 1) new-val acc1 acc2))))
|
|
|
|
(define (f2 n)
|
|
(if (< n 3)
|
|
n
|
|
(f-iter (- n 3) (f2 2) (f2 1) (f2 0))))
|
|
; these are just 2,1,0 respectively, but I felt this looks cleaner |